7 React Hooks You
Should Know
September 1, 2024 · 5 reading minutes
The most essential React hooks every frontend developer should know. From state management with useState to performance optimization with useMemo and useCallback, you'll understand how these hooks can transform the way you build React apps. You'll learn their use cases, practical examples, and best practices for implementing each of them in your projects.
Introduction to React Hooks
React has revolutionized the way we build user interfaces, and one of the most powerful innovations it has brought is hooks. Introduced in version 16.8, hooks allow developers to use state and other React features in functional components, something that was previously only possible with class components.
For any developer working with React, understanding and mastering hooks is essential. Not only do they simplify code, but they also allow you to create more efficient and maintainable applications. In this article, we'll look at how these hooks can transform the way you develop your applications.
By the end of this read, you'll be equipped with the knowledge to apply these hooks in your projects, improving your workflow and taking your React development skills to the next level.
1. useState: Local State Management
This is a hook that allows you to add state to a functional component. It returns a pair of values: the current state and a function to update it.
2. useEffect: Side Effects and Lifecycle
This hook is used to perform side effects on functional components, such as subscribing to services, making data requests, or manipulating the DOM. It runs after the component is rendered.
3. useContext: Sharing Global State
Allows access to the React context, which allows values (such as a theme or the authenticated user) to be shared between components without having to manually pass props through each level.
4. useReducer: Handling Complex State
This is similar to useState, but is useful for handling complex state or when state updates depend on a flow of actions. It uses a reducer to handle state updates based on the dispatched action.
5. useRef: References and Value Persistence
It provides a way to access DOM elements directly or save mutable values that do not require re-rendering the component when they change. It is also useful for keeping references to previous state values.
6. useMemo: Optimizing Expensive Calculations
This hook is used to memorize expensive calculations or functions to avoid recalculating them on every render, thus improving performance. It only recalculates the memorized value when its dependencies change.
7. useCallback: Memorize Functions for Performance
Memorize functions to avoid recreating them on every render. Useful when passing functions as props to child components that rely on the function reference to avoid unnecessary rendering.
Conclusions
React hooks have become indispensable tools for any developer who wants to create modern and efficient frontend applications. Throughout this article, we've explored seven of the most important hooks, each offering unique capabilities ranging from state management to performance optimization. By understanding and applying hooks like useState, useEffect, and useContext, you can simplify your components' logic, make your code more readable, and maintain a shared state without complications.
Additionally, hooks like useMemo and useCallback allow you to take your applications to the next level in terms of performance, avoiding unnecessary rendering and calculations. useReducer, on the other hand, provides you with a more structured way of handling complex states, especially useful in larger applications or with advanced state logic.
However, it's important to remember that the power of hooks also requires responsible use. Using them appropriately and in the right contexts ensures that your code remains clean and maintainable. With the knowledge you've gained about these hooks, you're better prepared to build more robust, efficient, and scalable React applications, taking full advantage of everything this library has to offer. As you continue to hone your use of hooks, you'll be strengthening your development skills and improving the quality of your projects.
Related articles
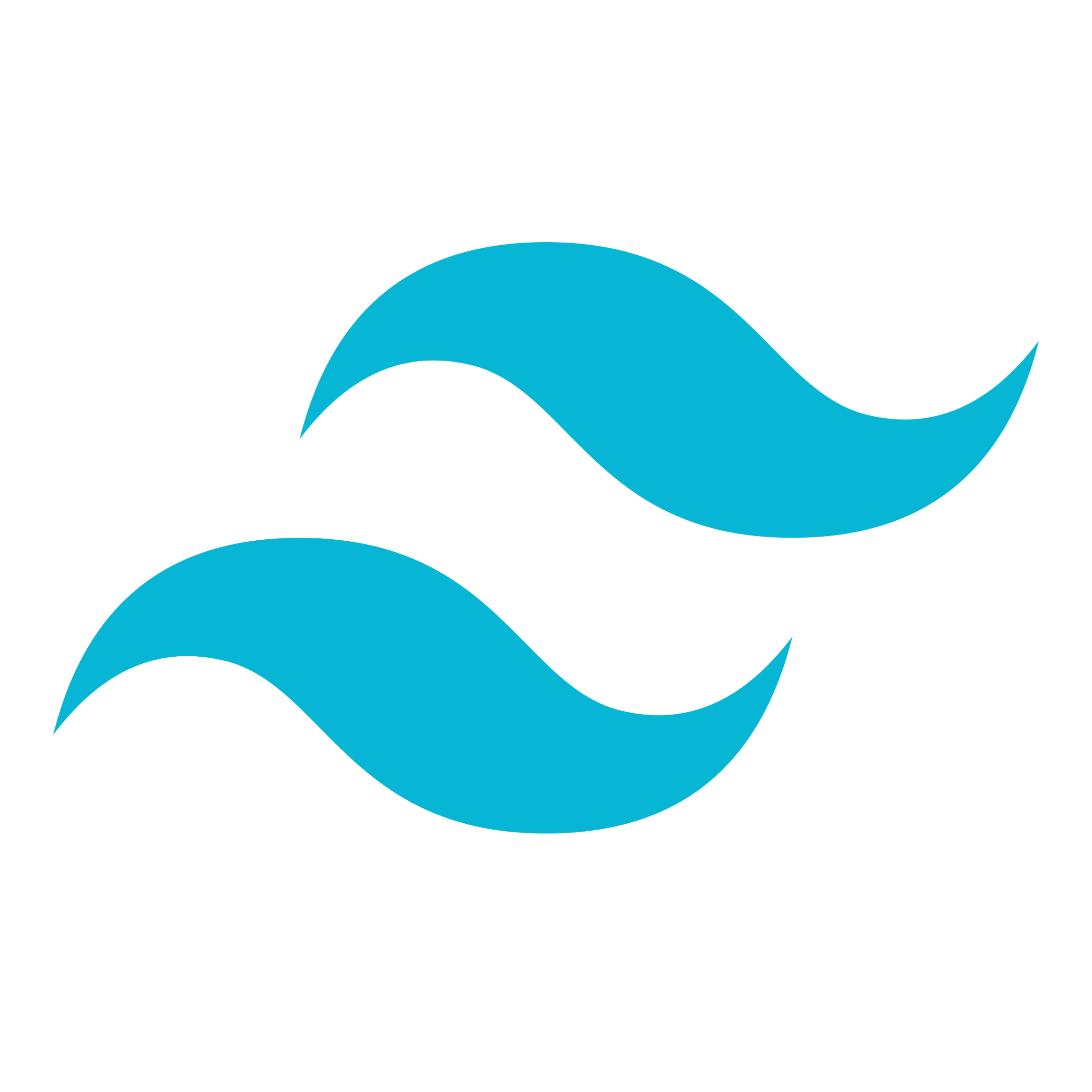
How to use Tailwind CSS for modern and responsive designs
Web Development
May 30, 2024
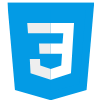
Best ways to center elements in CSS
Web Development
March 5, 2024
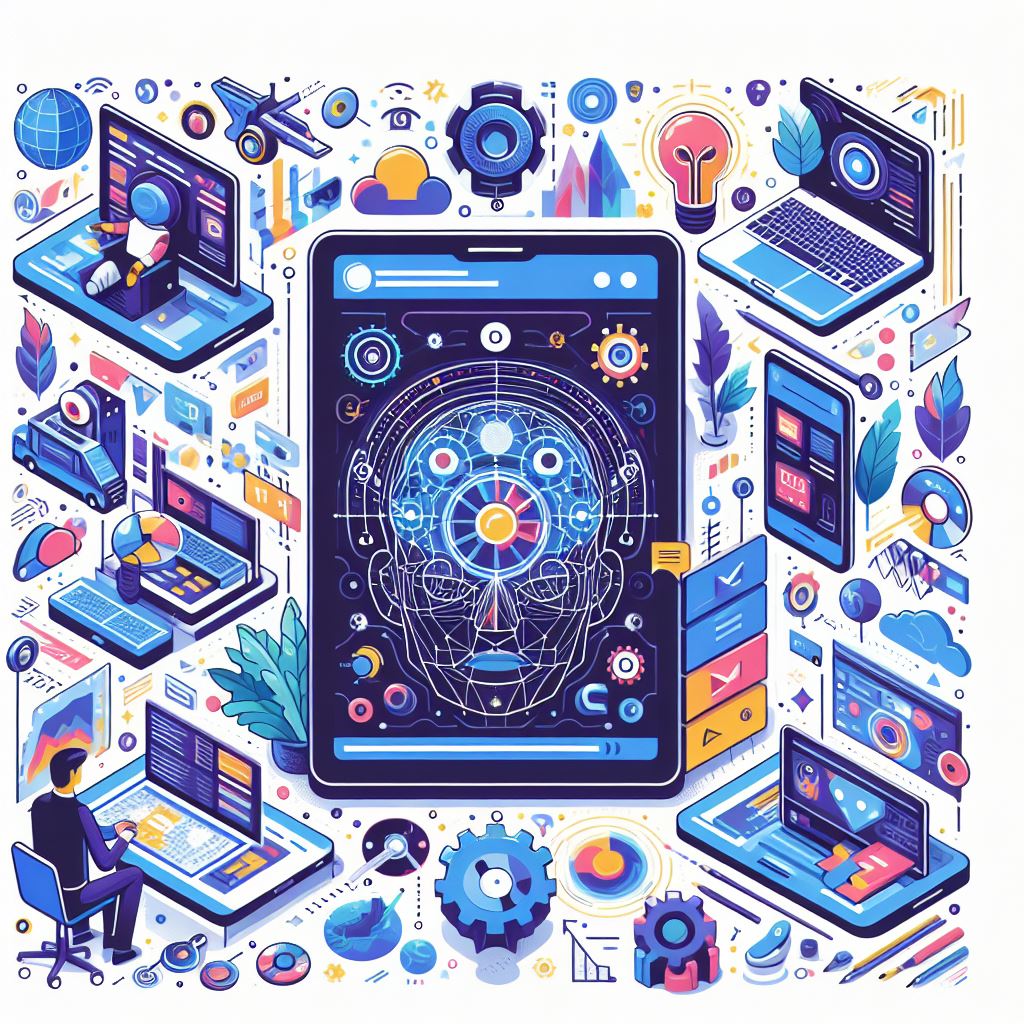
Exploring the Future Unveiling the Key Web Design Trends That Will Dominate 2024
Web design
December 30, 2023
.png)
How to Develop a Website from Scratch, From Idea to Success
Web Development
December 28, 2023
.jpg)
Building your Website from Scratch with Next.js. A React-Powered Development Journey
Web Development
December 28, 2023
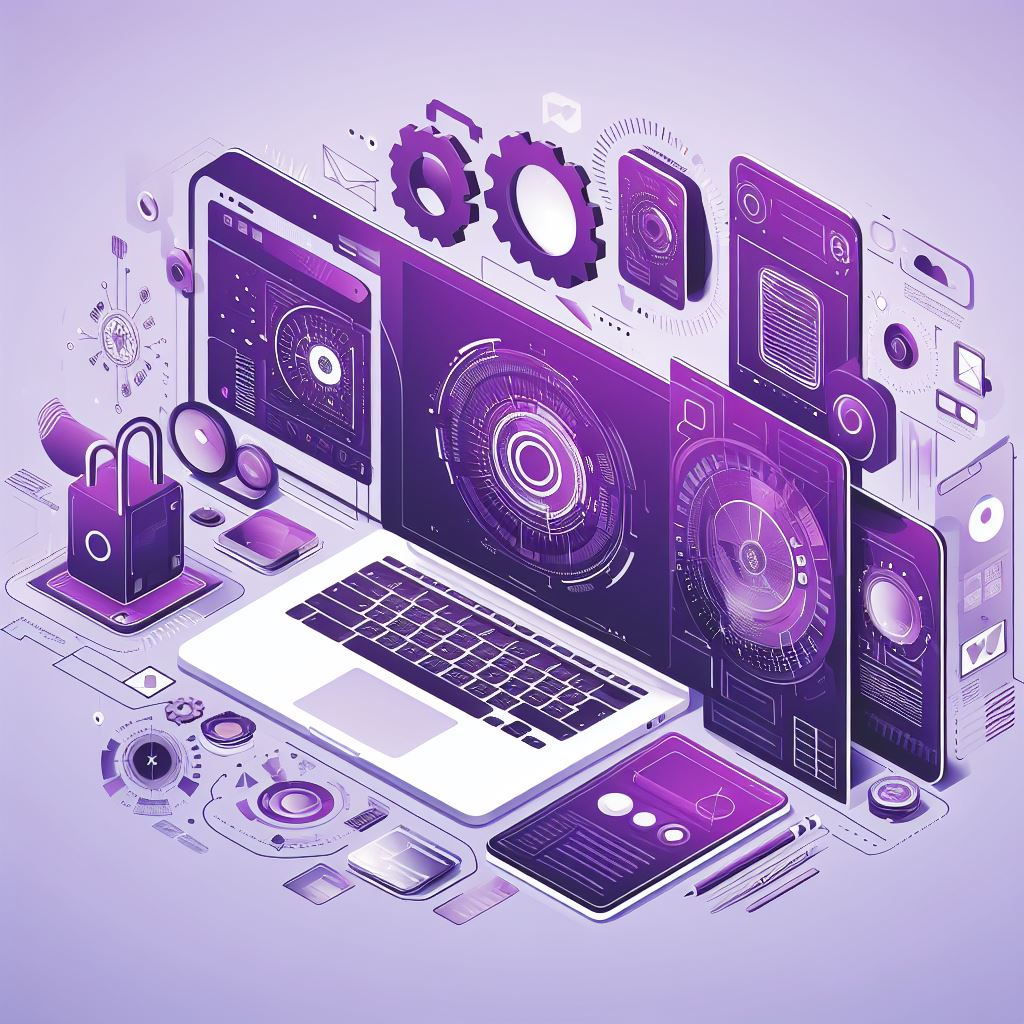
The New Technologies of Web Development, Boosting the Digital Experience
Web Development
November 24, 2023
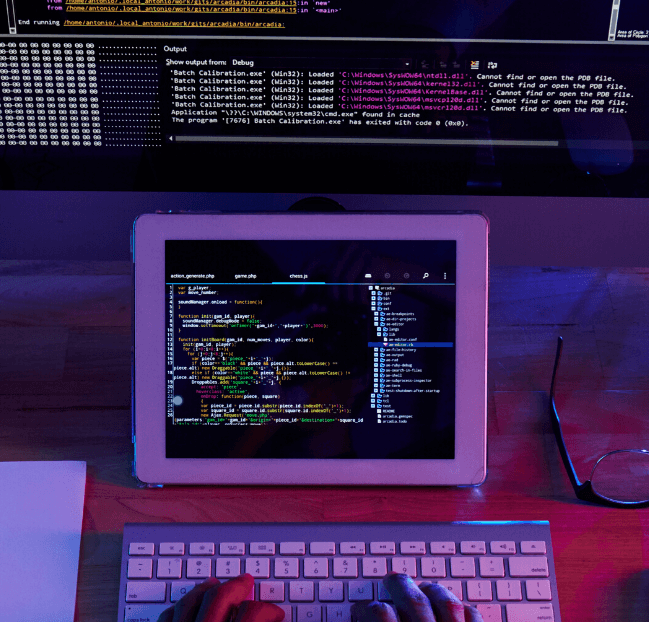
Motivation in Learning Web Development
Web Development
November 10, 2023
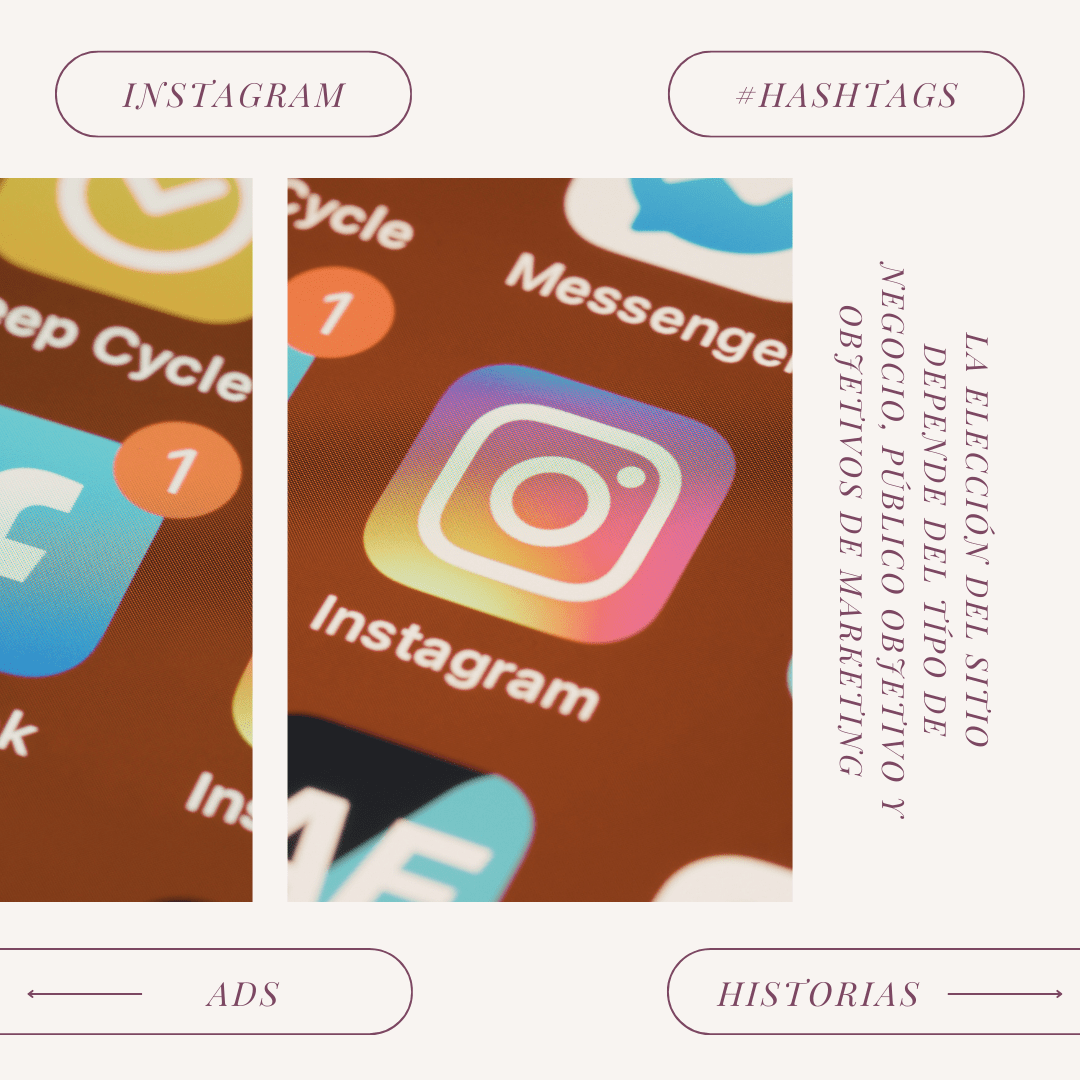
Choosing the Right Social Media Platforms for Your Business: Tips and Strategies
CateWebs Digital Portal
November 4, 2023
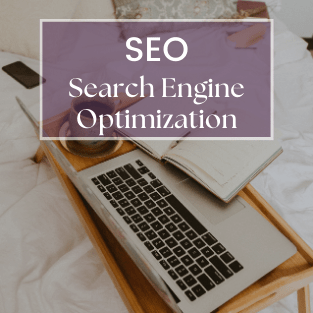
Will SEO still be relevant in 2024? Discover key trends
CateWebs Digital Portal
October 29, 2023
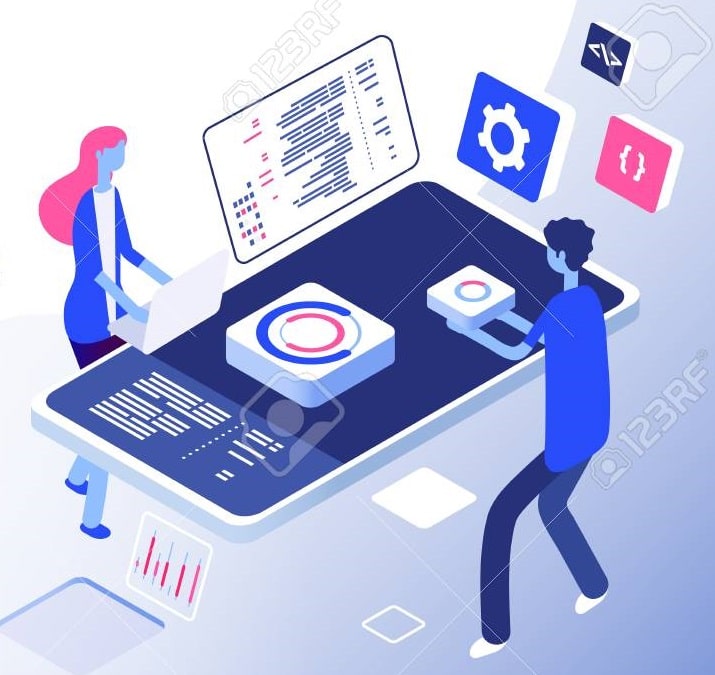
The Fascinating Web World of Frontend Development
Web Development
October 26, 2023